Here, We provide OOP GTU Paper Solution Winter 2022. Read the Full OOP GTU paper solution given below.
Object Oriented Programming-1 GTU Old Paper Winter 2022 [Marks : 70] : Click Here
(a) Discuss significance of byte code.
Bytecode is the intermediate code that is generated by the Java compiler and executed by the Java Virtual Machine (JVM). Here are some of the significant benefits of bytecode in the context of Java:
- Portability: Java bytecode is platform-independent, which means that it can be executed on any platform that has a JVM installed
- Security: Bytecode provides an additional layer of security as it can be executed within a sandbox environment. This sandbox ensures that the bytecode cannot access system resources directly, which helps prevent malicious code from damaging the system.
- Performance: While bytecode requires an additional step of interpretation or compilation by the JVM, it can be optimized for performance during this step. Additionally, the JVM can dynamically adapt its execution to improve performance based on the current system load and available resources.
- Maintainability: Bytecode can be easily decompiled back into source code, which can help developers understand and modify existing code.
(b) Explain Java garbage collection mechanism.
In Java, garbage collection is an automatic memory management mechanism that frees up memory that is no longer needed by the program.
It works by periodically scanning the heap, which is the region of memory where objects are stored, and identifying objects that are no longer being referenced by the program. These objects are then marked for garbage collection and their memory is released.
The garbage collection process is automatic and runs in the background without any intervention from the programmer.
Java uses a mark and sweep algorithm to identify objects that are no longer being referenced by the program. This algorithm works by first marking all objects that are still being referenced and then sweeping through the heap and releasing the memory of any objects that were not marked.
(c) List OOP characteristics and describe inheritance with examples.
Object-Oriented Programming (OOP) is a programming paradigm that focuses on creating objects that can interact with one another to solve problems. Here are the main characteristics of OOP:
- Encapsulation: The ability to hide implementation details within a class, and only expose relevant methods and properties to the outside world.
- Inheritance: The ability to create new classes based on existing classes, inheriting their properties and behavior.
- Polymorphism: The ability to use a single interface to represent multiple types of objects.
- Abstraction: The ability to focus on the essential features of an object, ignoring its implementation details.
Inheritance is a key aspect of OOP that allows developers to create new classes based on existing classes, inheriting their properties and behavior.
Inheritance creates a parent-child relationship between classes, where the child class inherits all the properties and methods of the parent class, and can also add new properties and methods of its own.
class Animal {
void makeSound() {
System.out.println("The animal makes a sound");
}
}
class Dog extends Animal {
void makeSound() {
System.out.println("The dog barks");
}
}
public class Main {
public static void main(String[] args) {
Animal animal = new Animal();
animal.makeSound(); // Output: The animal makes a sound
Dog dog = new Dog();
dog.makeSound(); // Output: The dog barks
}
}
In this example, the Animal
class is the parent class, and the Dog
class is the child class that extends the Animal
class. The Dog
class overrides the makeSound
method of the Animal
class to provide its own implementation.
When the makeSound
method is called on the animal
object, the output is "The animal makes a sound"
. When the makeSound
method is called on the dog
object, the output is "The dog barks"
.
(a) Explain constructor with the help of an example.
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
System.out.println("A new person has been created!");
}
}
In this example, we define a class called "Person" with two private attributes: "name" and "age". We also define a constructor for the class that takes two arguments, “name” and “age“.
Inside the constructor, we use the "this" keyword to refer to the current instance of the class and assign the values of the "name" and "age" parameters to the corresponding attributes of the instance. We also print a message to indicate that a new person has been created.
When a new instance of the Person class is created using the constructor, the “name” and “age” values are set and the message is printed to the console. For example:
Person john = new Person("John", 25);
This code creates a new instance of the Person class with the name “John” and age “25”, and the message “A new person has been created!” is printed to the console.
(b) List out different methods available for String class in java and explain
any two with proper example.
The String class in Java has a large number of methods available for manipulating and analyzing strings. Here are some of the most commonly used methods:
length()
: This method returns the length of the string, which is the number of characters in the string.
charAt(int index)
: This method returns the character at the specified index in the string. The index is zero-based, meaning that the first character in the string has an index of 0.
concat(String str)
: This method concatenates the specified string to the end of the original string and returns a new string that represents the concatenation.
substring(int beginIndex)
: This method returns a substring of the original string, starting at the specified index and continuing to the end of the string.
indexOf(char c)
: This method returns the index of the first occurrence of the specified character in the string, or -1 if the character is not found.
replace(char oldChar, char newChar)
: This method replaces all occurrences of the specified old character with the specified new character and returns a new string that represents the modified string.
String str = "hello";
int len = str.length(); // len is 5
String str = "hello";
char c = str.charAt(1); // c is 'e'
(c) Explain all access modifiers and their visibility as class members.
In object-oriented programming, access modifiers are keywords used to set the accessibility or visibility of class members (fields, methods, and inner classes) in a class. There are four access modifiers in Java:
- Public: Public members are accessible to all classes, regardless of the package they belong to. Public members can be accessed by any other class in the same program.
- Private: Private members are only accessible within the same class. They cannot be accessed by any other class, even if it belongs to the same package.
- Protected: Protected members are accessible within the same class, as well as any subclass that extends the class. They cannot be accessed by any other class, including those in the same package.
- Default (also known as package-private): Members with no access modifier specified are only accessible within the same package. They cannot be accessed by any other class outside the package.
Here’s a table that summarizes the visibility of class members based on their access modifiers:
Access Modifier | Visibility |
---|---|
Public | Accessible to all classes in any package |
Private | Accessible only within the same class |
Protected | Accessible within the same class and any subclass |
Default | Accessible only within the same package |
(c) Compare String with StringBuffer. Also, write a program to count the
occurrence of a character in a string.
String is an immutable class, which means that once a string object is created, its value cannot be changed. This means that any operation that modifies a string actually creates a new string object.
This can be efficient for operations like concatenation, but can lead to unnecessary memory allocation in situations where a large number of string objects are created.
StringBuffer, on the other hand, is a mutable class, which means that its value can be changed after it is created.
StringBuffer objects are generally more efficient than String objects for operations that involve a large number of modifications, because they can be modified in place without the need to create new objects.
public class CharCount {
public static int countOccurrences(String str, char c) {
int count = 0;
for (int i = 0; i < str.length(); i++) {
if (str.charAt(i) == c) {
count++;
}
}
return count;
}
public static void main(String[] args) {
String str = "hello world";
char c = 'l';
int count = countOccurrences(str, c);
System.out.println("The character '" + c + "' occurs " + count + " times in the string '" + str + "'");
}
}
Output : The character ‘l’ occurs 3 times in the string ‘hello world’
In this program, we define a static method called “countOccurrences” that takes two arguments: a String object and a character. The method uses a for loop to iterate over the characters in the string and counts the occurrences of the character by comparing each character to the target character.
(a) What is the final class? Why they are used?
A Final class is a class that cannot be subclassed, which means that no other class can inherit from it.
There are a few reasons why you might want to make a class final:
- Security: If a class contains sensitive information or functionality that should not be modified or extended, making it final can help prevent unauthorized access or modification.
- Efficiency: Final classes are often used to improve performance in heavily used or performance-critical code, since the compiler can make certain optimizations that would not be possible if the class could be subclassed.
- Clarity: Final classes can also be used to make code more clear and readable by indicating that a class is not intended to be subclassed, which can help prevent errors or confusion.
(b) Write exception handling mechanisms in JAVA.
In Java, exception handling mechanisms are used to handle errors and exceptions that may occur during the execution of a program.
The main goal of exception handling is to prevent the program from crashing due to unexpected errors and to provide a way to gracefully recover from errors.
There are two types of exceptions in Java: checked exceptions and unchecked exceptions. Checked exceptions are checked at compile time, while unchecked exceptions are not checked at compile time.
Here is an example of how to use exception handling in Java:
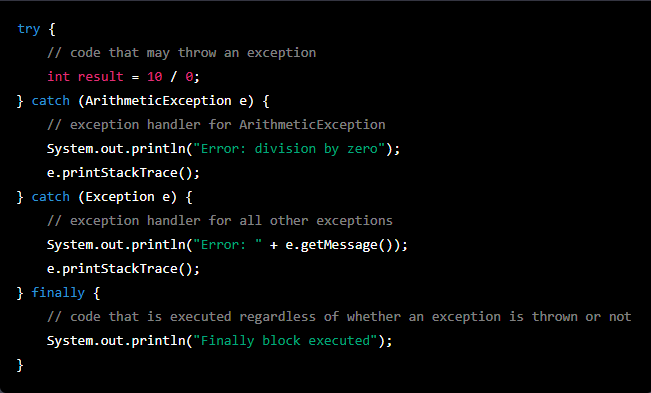
In this example, we use a try block to enclose the code that may throw an exception. If an exception is thrown, one of the catch blocks will handle the exception based on its type. In this case, we have a catch block for ArithmeticException, which is thrown when we try to divide by zero. We also have a catch block for Exception, which will handle all other types of exceptions that may occur.
The finally block is executed regardless of whether an exception is thrown or not. It is typically used to perform cleanup tasks or release resources that were acquired in the try block.
(c) Explain Overloading and Overriding with example.
Overloading : –
Overloading and overriding are two important concepts in Java that are used to achieve polymorphism, which allows a single method or class to have multiple behaviors based on the context in which it is used.
Overloading is the practice of using the same method name with different parameters or argument lists. When a method is overloaded, the compiler determines which version of the method to call based on the number and types of arguments passed to it.
Here is Example of Method Overloading
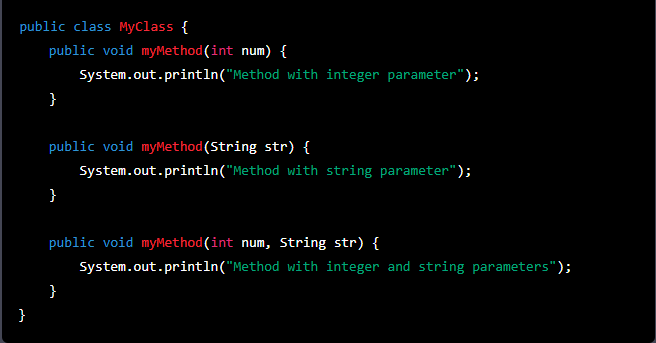
In this example, we have three versions of the myMethod
method, each with a different parameter list. When we call
, the compiler will determine which version of the method to call based on the type and number of arguments passed to it. myMethod
Overriding : –
Overriding, on the other hand, is the practice of providing a new implementation for a method in a subclass that is already defined in the parent class. When a method is overridden, the version of the method in the subclass is called instead of the version in the parent class.
Here is Example of Method Overriding
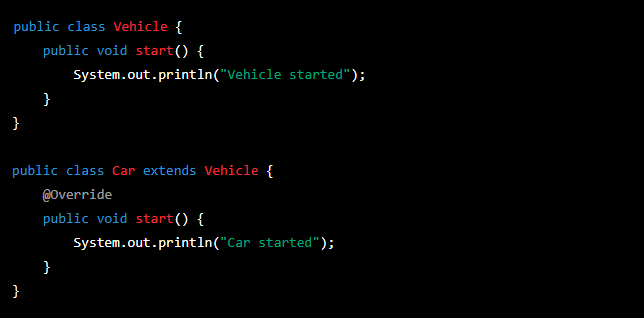
In this example, we have a parent class Vehicle
with a method start, and a subclass Car
that overrides the start method with a new implementation. When we create a Car
object and call the start method, the version of the method in the Car
class is called instead of the version in the Vehicle
class.
(a) How can you create packages in Java?
In Java, a package is a way to organize related classes, interfaces, and other resources. A package can contain other packages and classes.
To create a package in Java, you can follow these steps:
- Create a new directory with the name of your package. The directory name should be the same as your package name, and each sub-package should be a subdirectory within the main package directory.
- Add a package statement at the top of each Java file that belongs to the package. The package statement should indicate the full name of the package, including any parent packages.
(b) What is Inheritance? List out the different forms of Inheritance and
explain any one with example.
Inheritance is a fundamental concept in object-oriented programming that allows a new class to be based on an existing class. The new class inherits the properties and behavior of the existing class and can add its own properties and behavior. Inheritance promotes code reuse and makes it easier to create and maintain complex programs.
There are different types of inheritance in Java:
- Single inheritance: A subclass inherits from a single parent class.
- Multilevel inheritance: A subclass inherits from a parent class, which in turn inherits from another parent class.
- Hierarchical inheritance: Multiple subclasses inherit from a single parent class.
- Multiple inheritance: A subclass inherits from multiple parent classes.
Java does not support multiple inheritance directly, but it allows multiple inheritance through interfaces, which are similar to abstract classes.
Here is an example of single inheritance in Java:
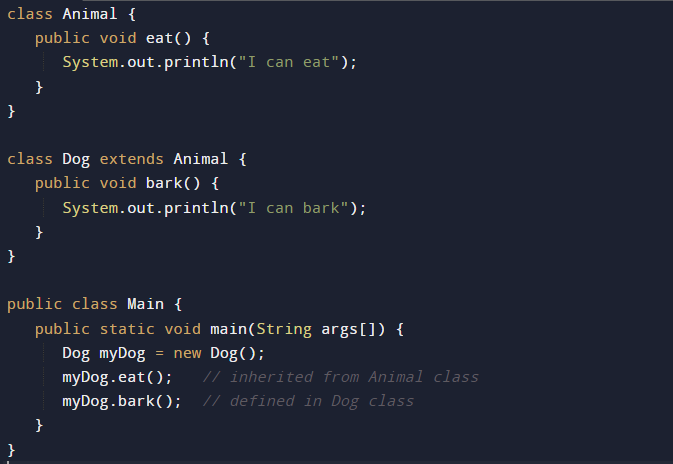
In this example, we have a parent class called “Animal” which has a method called “eat”. We then define a subclass called “Dog” which extends the “Animal” class and has a method called “bark”. In the main method, we create an object of the “Dog” class and call both the “eat” and “bark” methods.
In this way, the subclass “Dog” inherits the “eat” method from the parent class “Animal” through single level inheritance, and also defines its own method “bark”. Single level inheritance allows us to reuse code from a parent class in a subclass, making our code more efficient and maintainable.
(c) Explain the words super, static, final and this with the help of an
example.
Super :-
“super” is a keyword in Java that is used to refer to the superclass of the current object. It is used to call the superclass constructor, access the superclass’s methods, or access the superclass’s variables.
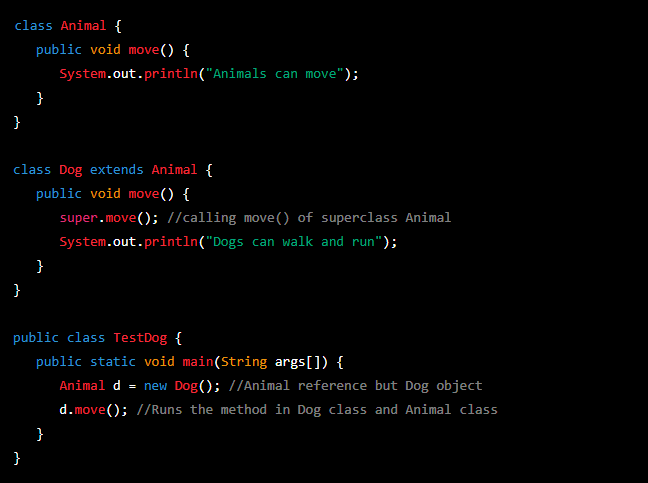
Static:-
“static” is a keyword in Java that is used to define a variable, method or block as a class-level entity. A static variable or method is associated with the class and not with any particular instance of that class. Static members are initialized only once when the class is loaded into memory.
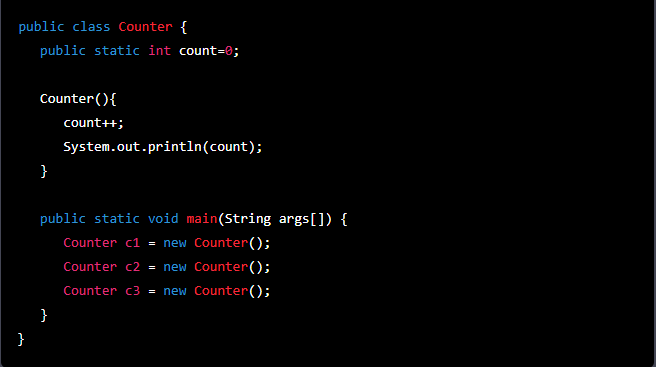
In the above example, count is a static variable of the class Counter
. When each object of Counter
is created, the constructor is called and the value of count
is incremented by 1. As count
is a static variable, it is associated with the class rather than with any particular instance of the class. Therefore, the value of count
is incremented for every object created, and the output is 1 2 3
.
Final :-
“final” is a keyword in Java that is used to make a variable, method or class unchangeable or constant. Once a final variable is initialized, its value cannot be changed. A final method cannot be overridden by a subclass, and a final class cannot be extended.
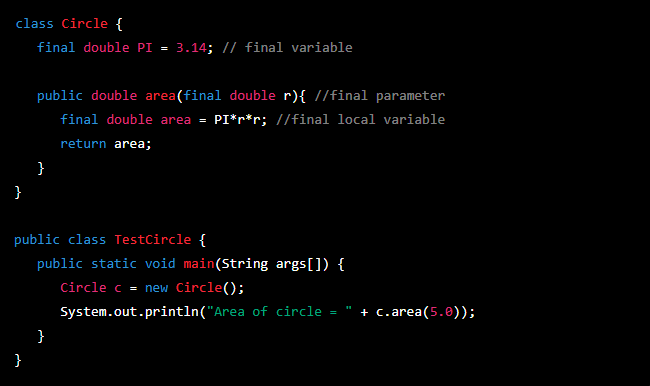
In the above example, PI
is a final variable that is initialized with the value of 3.14. Once initialized, the value of PI
cannot be changed. area()
method takes a final parameter r
, which means that the value of r
cannot be changed inside the method. The local variable area
is also declared as final, which means its value cannot be changed once initialized.
This :-
“this” is a keyword in Java that refers to the current object. It can be used to refer to instance variables and instance methods of the current class. It is also used to call one constructor from another constructor in the same class.
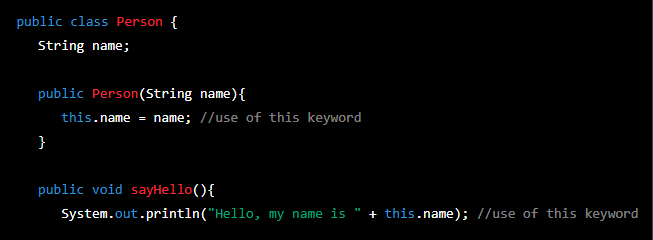
(a) List out various layout panes in JavaFX.
JavaFX provides several built-in layout panes that allow developers to organize UI components on a screen in a structured way. Some of the commonly used layout panes in JavaFX are:
- BorderPane: A layout pane that divides the screen into five regions: top, bottom, left, right, and center.
- HBox: A layout pane that arranges its child nodes horizontally in a single row.
- VBox: A layout pane that arranges its child nodes vertically in a single column.
- StackPane: A layout pane that stacks its child nodes one on top of another.
- GridPane: A layout pane that arranges its child nodes in a grid of rows and columns.
- FlowPane: A layout pane that arranges its child nodes in a flow from left to right or top to bottom.
- TilePane: A layout pane that arranges its child nodes in a grid, with each cell being the same size.
- AnchorPane: A layout pane that allows you to anchor child nodes to the edges of the screen.
- Pane: A generic layout pane that allows you to place child nodes at arbitrary locations within the pane.
(b) Explain the architecture of JavaFX.
JavaFX is a platform for building rich and interactive graphical user interfaces (GUIs) in Java. It was introduced in 2008 as the successor to the Swing toolkit, and is built on top of the Java SE platform. Here is a brief overview of the architecture of JavaFX:
- Scene Graph – The Scene Graph is the core of the JavaFX architecture. It is a hierarchical structure of nodes, where each node represents an element in the GUI, such as a button, label, or text field. The Scene Graph provides a declarative and customizable way to create and layout the user interface.
- CSS Styling – JavaFX allows developers to style the user interface using CSS (Cascading Style Sheets). CSS is a powerful styling language that separates the presentation of the user interface from the logic of the application. Developers can create custom styles for each node in the Scene Graph, or use pre-defined styles from the JavaFX library.
- Event Handling – JavaFX provides a rich set of event handlers that allow developers to handle user input, such as mouse clicks and keyboard input. Event handling in JavaFX is based on the observer pattern, where event sources notify event listeners of user actions.
- Animation – JavaFX provides a powerful animation framework that allows developers to create complex animations and transitions in the user interface. The animation framework is based on the timeline and keyframe concepts, where developers can define a sequence of keyframes that define the animation.
- Media Support – JavaFX provides built-in support for playing media, such as video and audio files. It supports a variety of media formats and provides a flexible media player API for controlling playback.
- 3D Graphics – JavaFX provides a powerful 3D graphics framework that allows developers to create and manipulate 3D objects in the user interface. The 3D graphics framework is based on the scene graph, and supports features such as lighting, textures, and materials.
(c) Discuss BufferedInputStream and BufferedOutputStream classes with
an example.
The BufferedInputStream and BufferedOutputStream classes are used in Java to improve the performance of input and output operations by buffering the data. Both classes are subclasses of FilterInputStream and FilterOutputStream, respectively.
BufferedInputStream reads data from an input stream and stores it in an internal buffer, allowing for efficient reading of data in larger chunks.
Similarly, BufferedOutputStream writes data to an output stream in buffered chunks, improving the performance of the output operation.
Here’s an example of how to use BufferedInputStream and BufferedOutputStream:
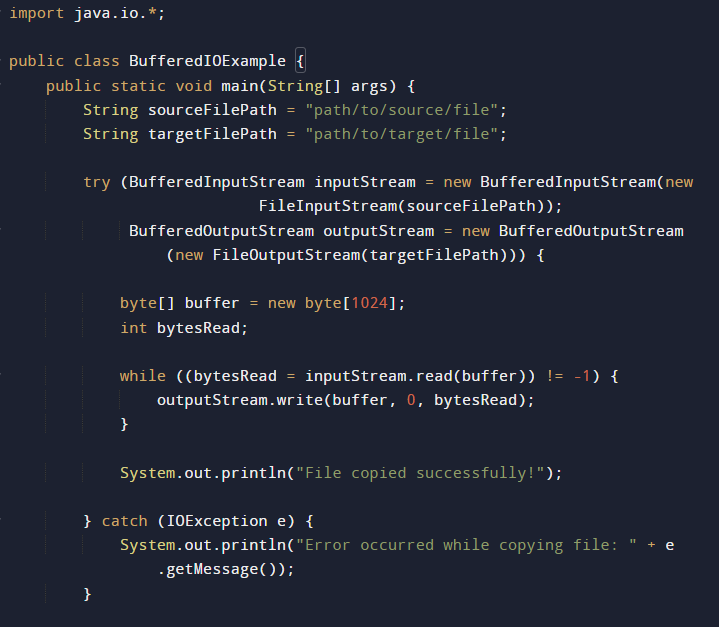
In the above example, we are copying data from the source file to the target file using BufferedInputStream and BufferedOutputStream. We create an instance of BufferedInputStream and BufferedOutputStream by wrapping them around FileInputStream and FileOutputStream, respectively.
We then create a buffer of size 1024 bytes and read data from the input stream in chunks of this size until all the data has been read. The read() method returns the number of bytes that have been read, which we pass to the write() method of the output stream to write the data to the target file.
(a) List out JavaFX UI controls and explain any one in detail.
JavaFX provides a wide range of UI controls that developers can use to create rich and interactive user interfaces. Some of the commonly used JavaFX UI controls are:
- Button: A control that represents a clickable button.
- Label: A control that displays a text label.
- TextField: A control that allows the user to input text.
- TextArea: A control that allows the user to input multi-line text.
- CheckBox: A control that represents a check box.
- RadioButton: A control that represents a radio button.
- ComboBox: A control that represents a drop-down list of options.
- ListView: A control that displays a list of items.
- TableView: A control that displays data in a tabular format.
- DatePicker: A control that allows the user to select a date.
(b) Demonstrate animation effect in JavaFX.
import ....
public class AnimationExample extends Application {
@Override
public void start(Stage primaryStage) {
Circle circle = new Circle(50, Color.BLUE);
StackPane root = new StackPane(circle);
Scene scene = new Scene(root, 200, 200);
TranslateTransition transition = new TranslateTransition(Duration.seconds(2), circle);
transition.setByX(100);
transition.setByY(100);
transition.setAutoReverse(true);
transition.setCycleCount(TranslateTransition.INDEFINITE);
transition.play();
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
(c) Create a class called Student. Write a student manager program to
manipulate the student information from files by using FileInputStream
and FileOutputStream.
import java.io.*;
import java.util.ArrayList;
import java.util.List;
public class Student {
private int id;
private String name;
private String address;
public Student(int id, String name, String address) {
this.id = id;
this.name = name;
this.address = address;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public String getAddress() {
return address;
}
}
class StudentManager {
private static final String FILE_NAME = "students.txt";
public static void main(String[] args) {
List<Student> students = new ArrayList<>();
students.add(new Student(1, "John Doe", "123 Main St"));
students.add(new Student(2, "Jane Doe", "456 Oak Ave"));
students.add(new Student(3, "Bob Smith", "789 Elm St"));
saveStudentsToFile(students);
List<Student> loadedStudents = loadStudentsFromFile();
System.out.println("Loaded students:");
for (Student student : loadedStudents) {
System.out.println(student.getId() + " " + student.getName() + " " + student.getAddress());
}
}
private static void saveStudentsToFile(List<Student> students) {
try (FileOutputStream fos = new FileOutputStream(FILE_NAME);
ObjectOutputStream oos = new ObjectOutputStream(fos)) {
oos.writeObject(students);
} catch (IOException e) {
e.printStackTrace();
}
}
private static List<Student> loadStudentsFromFile() {
List<Student> students = new ArrayList<>();
try (FileInputStream fis = new FileInputStream(FILE_NAME);
ObjectInputStream ois = new ObjectInputStream(fis)) {
students = (List<Student>) ois.readObject();
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
return students;
}
}
(a) What is Java Collection?
In Java, a collection is a group of objects that can be treated as a single entity. Java provides a framework of classes and interfaces called the Java Collections Framework that makes it easy to work with collections of objects.
The Java Collections Framework provides several interfaces and classes that represent different types of collections, such as lists, sets, maps, and queues. Each collection type has its own set of characteristics and can be used for different purposes.
For example, a List
is an ordered collection that allows duplicates, while a Set
is an unordered collection that does not allow duplicates. A Map
is a collection that maps keys to values, and a Queue
is a collection that represents a waiting line or a sequence of elements to be processed in a particular order.
(b) List out methods of Iterator and explain it.
In Java, the Iterator interface provides a way to iterate over a collection of elements, such as those in a List or Set, in a safe and efficient manner. It has several methods that allow you to manipulate the collection while iterating over it. Here are the methods of the Iterator interface and their explanations:
- hasNext() – Returns true if there are more elements in the collection, false otherwise.
- next() – Returns the next element in the collection and advances the iterator.
- remove() – Removes the last element returned by the iterator from the underlying collection. This method is optional and may throw an UnsupportedOperationException if the collection does not support removal.
Here is an example of how to use an Iterator to iterate over a List of integers and remove elements that meet a certain condition:
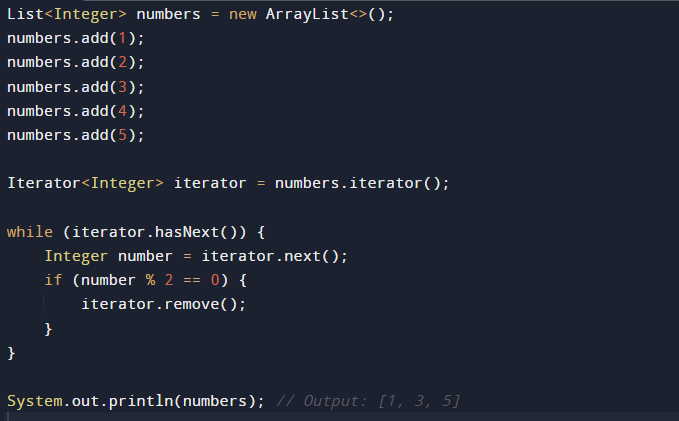
In this example, we create a List of integers and add five numbers to it. We then create an Iterator object using the iterator() method of the List interface.
We use a while loop to iterate over the list, calling hasNext() to check if there are more elements and next() to get the next element. If the current element is even, we remove it using the remove() method of the Iterator interface. Finally, we print the contents of the list after removing all even numbers.
(c) Explain Set and Map in Java with example.
In Java, Set and Map are two common interfaces that are used to store and manipulate collections of data.
Set :
A Set is a collection of unique elements, which means that it does not allow duplicates. The elements in a Set are not ordered, and their positions can change during runtime. The Set interface is implemented by several classes in Java, such as HashSet, TreeSet, and LinkedHashSet.
Here is an example of using a HashSet to store a set of unique strings:
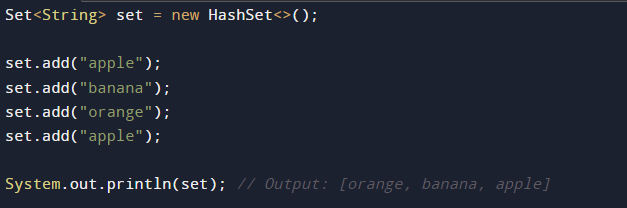
In this example, we create a HashSet and add four strings to it. Because “apple” appears twice in the list, only one of them is added to the set, resulting in a set of three unique elements. The output shows that the elements in the set are not ordered.
Map :
A Map is a collection of key-value pairs, where each key is associated with a unique value. The keys in a Map are unique, but the values can be duplicated. The Map interface is implemented by several classes in Java, such as HashMap, TreeMap, and LinkedHashMap.
Here is an example of using a HashMap to store a set of key-value pairs:
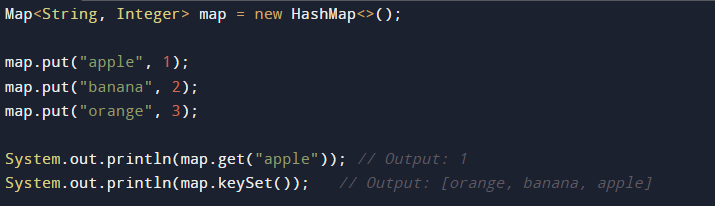
In this example, we create a HashMap and add three key-value pairs to it. We can retrieve the value associated with a particular key using the get() method, which returns the value 1 for the key “apple”. We can also retrieve a set of all the keys in the map using the keySet() method, which returns a set containing “apple”, “banana”, and “orange”.
(a) What is Vector class?
In Java, a Vector is a class that represents a dynamic array, which means that its size can change during runtime. It is similar to an ArrayList, but it is synchronized, which means that it is thread-safe, and multiple threads can access it at the same time without any issues.
The Vector class provides several methods to manipulate and access elements of the array, such as add(), remove(), get(), set(), size(), and more. It can be used to store objects of any type, and it automatically resizes itself as elements are added or removed.
However, it is important to note that the Vector class is considered to be less efficient than the ArrayList class because of its synchronized nature. Therefore, it is recommended to use ArrayList instead of Vector in most cases, unless synchronization is specifically required.
(b) Describe with diagram the life cycle of Thread.
The life cycle of a thread in Java can be described using the following diagram:
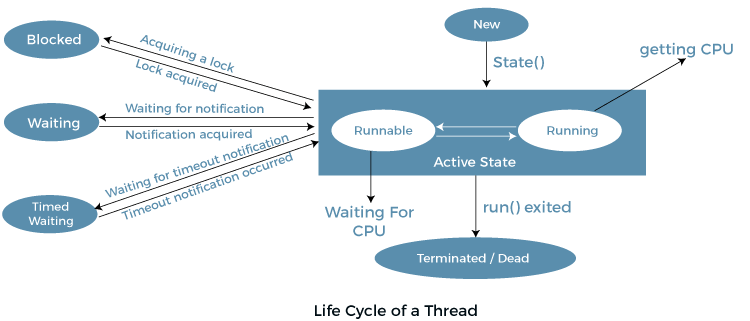
- New: The thread is in the new state when it is created, but it has not yet started to execute.
- Runnable: When the start() method is called on a thread object, it enters the runnable state. In this state, the thread is ready to run, but the operating system has not yet assigned a processor to it.
- Running: When the operating system assigns a processor to the thread, it enters the running state. The thread executes the code in its run() method.
- Blocked: A thread enters the blocked state when it is waiting for a monitor lock to be released, such as when it is waiting to enter a synchronized block.
- Waiting: A thread enters the waiting state when it is waiting for another thread to perform a particular action, such as when it calls the wait() method.
- Timed Waiting: A thread enters the timed waiting state when it is waiting for a specified period of time, such as when it calls the sleep() method.
- Terminated: When a thread completes its run() method or throws an unhandled exception, it enters the terminated state and cannot be restarted.
This life cycle diagram of a thread in Java illustrates how a thread can transition from one state to another based on various conditions and events.
(c) Explain synchronization in Thread with suitable example.
In Java, synchronization is the process of controlling access to shared resources such as variables, objects, and methods in a multithreaded environment. When multiple threads access the same shared resource concurrently, synchronization ensures that only one thread can access the resource at a time, preventing data inconsistency and race conditions.
A common way to achieve synchronization in Java is by using the synchronized keyword. When a method or a block of code is marked as synchronized, only one thread can execute it at a time, and other threads must wait until the synchronized block is released.
Here is an example of using synchronization to ensure thread safety:
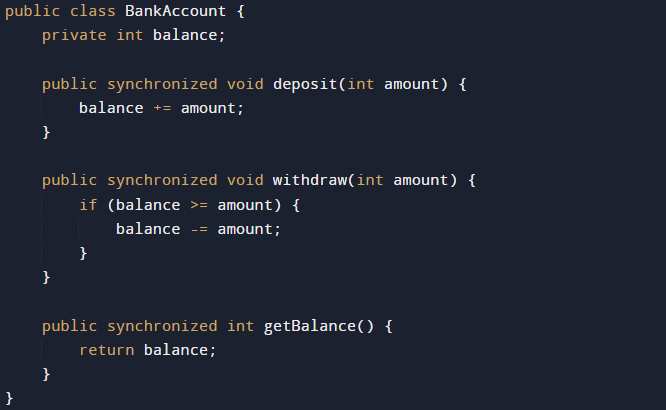
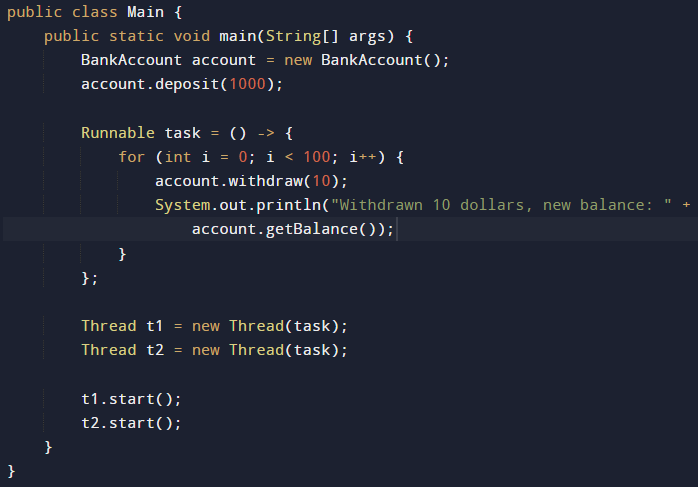
In this example, we have a BankAccount class with deposit(), withdraw(), and getBalance() methods. All of these methods are marked as synchronized, which means that only one thread can access them at a time.
In the main() method, we create two threads and pass a task to them that repeatedly withdraws 10 dollars from the account. Because the withdraw() method is synchronized, only one thread can withdraw money from the account at a time, preventing data inconsistency and race conditions.
By using synchronization in this way, we ensure that our BankAccount class is thread-safe and can be used in a multithreaded environment without any issues.
Read More : DBMS GTU Paper Solution Winter 2021
Read More : DS GTU Paper Solution Winter 2021
“Do you have the answer to any of the questions provided on our website? If so, please let us know by providing the question number and your answer in the space provided below. We appreciate your contributions to helping other students succeed.