Here, We provide OOP GTU Paper Solution Summer 2022. Read the Full OOP GTU paper solution given below.
Object Oriented Programming-1 GTU Old Paper Summer 2022 [Marks : 70] : Click Here
(a) List out features of Java. Explain any two features.
Java is a popular programming language that was developed in the mid-1990s by Sun Microsystems (now owned by Oracle Corporation). Some of the key features of Java are:
- Platform Independence
- Object-oriented
- Security
- Portability
- Garbage Collection
Two key features of Java are:
- Platform Independence – Java code can run on any platform that supports the Java Virtual Machine (JVM). This makes it easy to develop and deploy software that can run on multiple platforms without the need for recompilation. For example, a Java program written on a Windows machine can be run on a Linux or Mac machine without any changes to the code.
- Object-oriented – Java is an object-oriented programming language, which means that it supports encapsulation, inheritance, and polymorphism. This makes it easy to write modular and reusable code. Object-oriented programming allows developers to break down complex problems into smaller, more manageable pieces. This makes it easier to develop, test, and maintain software over time.
(b) Write a single program which demonstrates the usage of following
keywords:
i) import, ii) new, iii) this, iv) break, v) continue
Show how to compile and run the program in java.
Here’s an example program that demonstrates the usage of the keywords import, new, this, break, and continue:
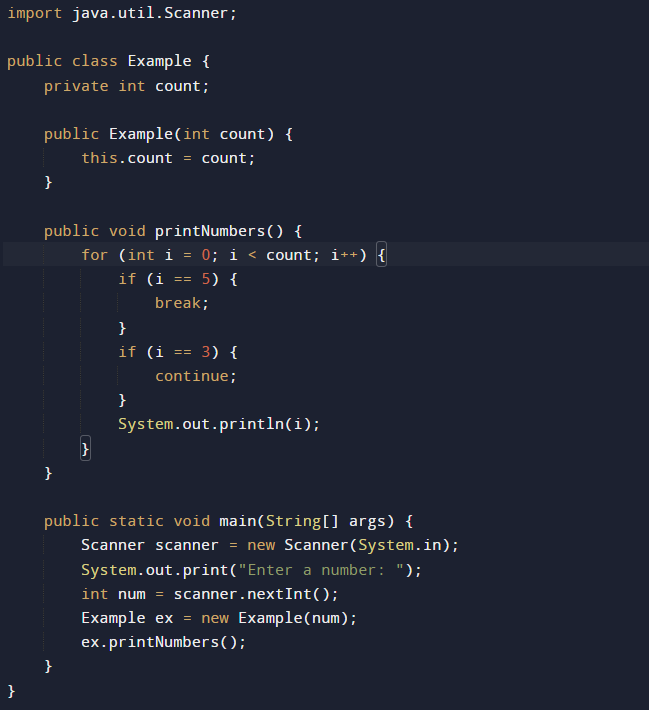
Explanation:
import java.util.Scanner;
– This line imports theScanner
class from thejava.util
package, which is used to read user input from the console.new Example(num);
– This line creates a new instance of theExample
class using thenew
keyword.this.count = count;
– This line uses thethis
keyword to refer to the current instance of theExample
class and assigns thecount
parameter to itscount
field.break;
– This keyword is used to break out of a loop or switch statement.continue;
– This keyword is used to skip to the next iteration of a loop.
To compile and run this program, follow these steps:
- Save the code as a file with a
.java
extension (e.g.Example.java
). - Open a command prompt or terminal window and navigate to the directory where the file is saved.
- Compile the program by typing
javac Example.java
and pressing Enter. - Run the program by typing
java Example
and pressing Enter.
(c) Demonstrate use of try-catch construct in case of hierarchical
Exception Handling. (i.e handling various exception belongs to the
exception hierarchy)
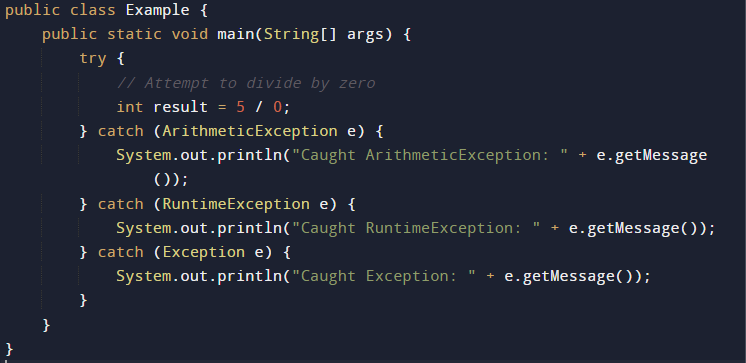
Explanation:
- In this example, we have intentionally tried to divide an integer by zero, which will throw an
ArithmeticException
. - We have used a
try
block to wrap the code that might throw an exception. - We have used multiple
catch
blocks to handle different types of exceptions. The firstcatch
block handlesArithmeticException
, the secondcatch
block handlesRuntimeException
, and the thirdcatch
block handles all other types of exceptions that are subclasses of theException
class. - If an exception is thrown, the appropriate
catch
block will be executed based on the type of exception. In this case, the firstcatch
block will be executed, and the message “Caught ArithmeticException: / by zero” will be printed to the console.
(a) Explain following Java keywords using appropriate examples:
i) static, ii) final, iii) super
Super :-
“super” is a keyword in Java that is used to refer to the superclass of the current object. It is used to call the superclass constructor, access the superclass’s methods, or access the superclass’s variables.
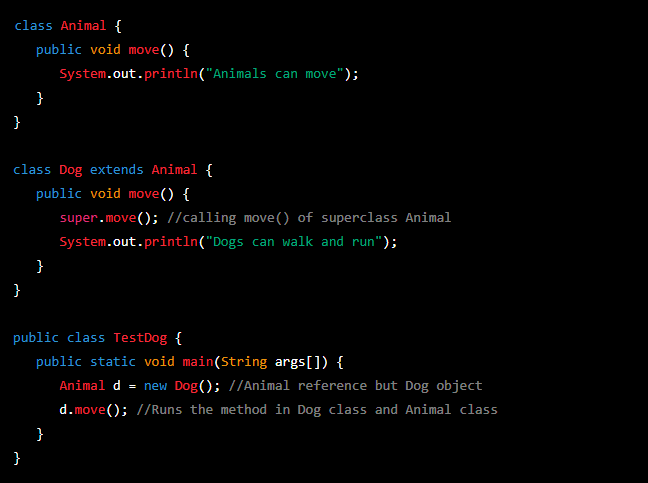
Static:-
“static” is a keyword in Java that is used to define a variable, method or block as a class-level entity. A static variable or method is associated with the class and not with any particular instance of that class. Static members are initialized only once when the class is loaded into memory.
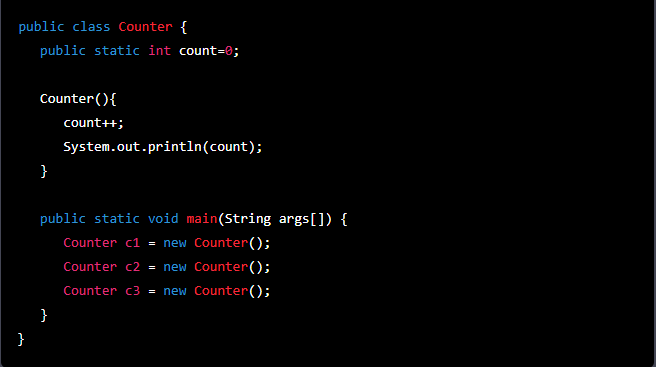
In the above example, count is a static variable of the class Counter
. When each object of Counter
is created, the constructor is called and the value of count
is incremented by 1. As count
is a static variable, it is associated with the class rather than with any particular instance of the class. Therefore, the value of count
is incremented for every object created, and the output is 1 2 3
.
Final :-
“final” is a keyword in Java that is used to make a variable, method or class unchangeable or constant. Once a final variable is initialized, its value cannot be changed. A final method cannot be overridden by a subclass, and a final class cannot be extended.
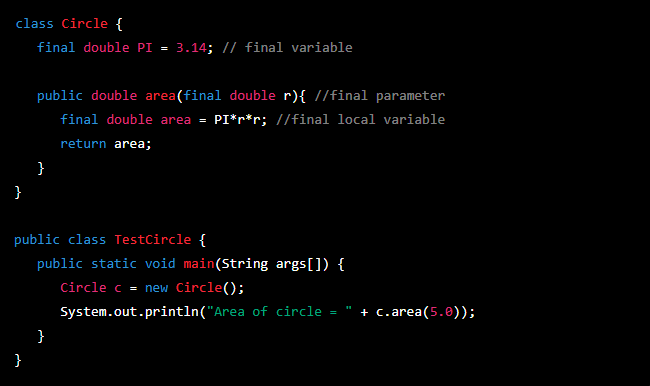
In the above example, PI
is a final variable that is initialized with the value of 3.14. Once initialized, the value of PI
cannot be changed. area()
method takes a final parameter r
, which means that the value of r
cannot be changed inside the method. The local variable area
is also declared as final, which means its value cannot be changed once initialized.
(b) Consider class A as the parent of class B. Explain among the
following which statement will show the compilation error.
i) A a = new A();
ii) A a = new B();
iii) B b = new A();
iv) B b = new B();
In this scenario, class B is a child class of class A, so it inherits all the members of class A. Now, let’s consider each statement and see which one will show a compilation error:
iii) B b = new A();
: This statement creates a new object of class A and assigns it to a variable of type B. This is not valid because A is the parent class and B is the child class, so an object of class A cannot be assigned to a variable of type B. This statement will result in a compilation error.
Therefore, statement (iii) B b = new A();
will show a compilation error.
(c) Write a java program to take infix expressions and convert it into
prefix expressions.
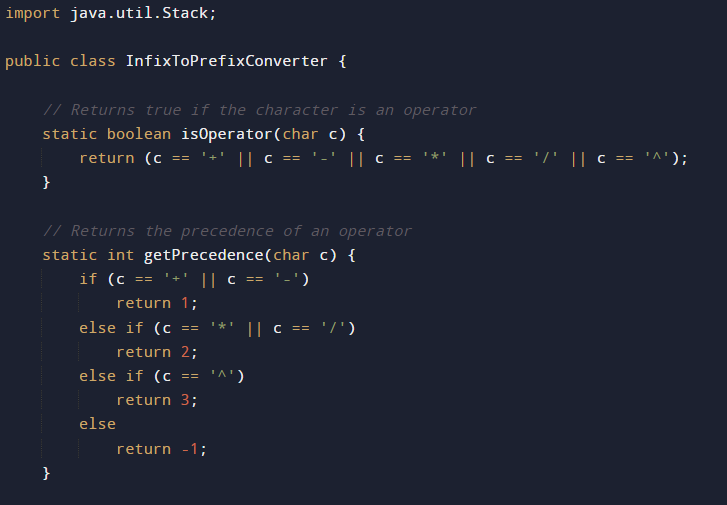
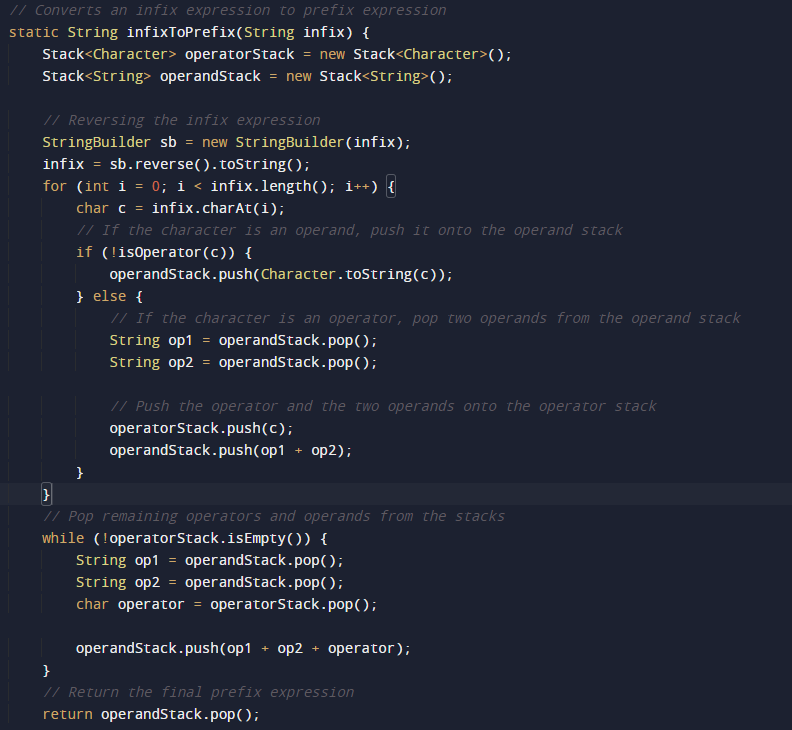
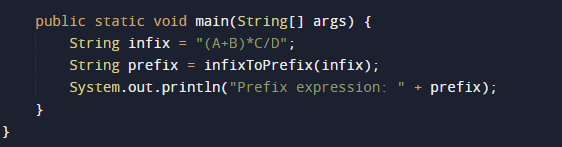
(c) Write a java program that evaluates a math expression given in string
form from command line arguments.
(a) Defines types of inheritance.
In Java, there are five types of inheritance:
- Single Inheritance: In single inheritance, a subclass extends a single superclass.
- Multilevel Inheritance: In multilevel inheritance, a subclass extends a superclass, which in turn extends another superclass, and so on.
- Hierarchical Inheritance: In hierarchical inheritance, multiple subclasses extend a single superclass.
- Multiple Inheritance: Multiple inheritance is a type of inheritance in which a subclass can have more than one superclass. However, Java does not support multiple inheritance directly.
- Hybrid Inheritance: Hybrid inheritance is a combination of multiple and hierarchical inheritance. It is achieved by combining two or more types of inheritance in a single program.
(b) Explain the following constructors using appropriate example:
i) Default constructor and Parameterised constructor
ii) Shallow copy and Deep copy constructor
i) Constructors:
Default constructor: A default constructor is a constructor with no parameters. If a class does not have any constructor defined, then a default constructor is automatically created by the compiler. The default constructor initializes the instance variables of the class with default values.
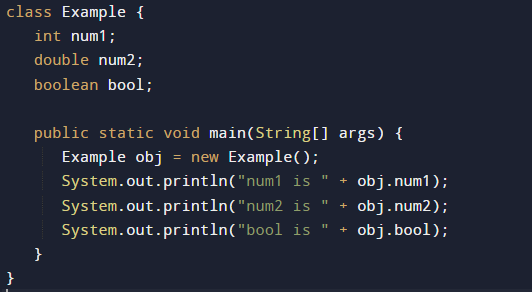
Parameterised constructor: A parameterized constructor is a constructor that takes parameters. It is used to initialize the instance variables of the class with the values passed as parameters.
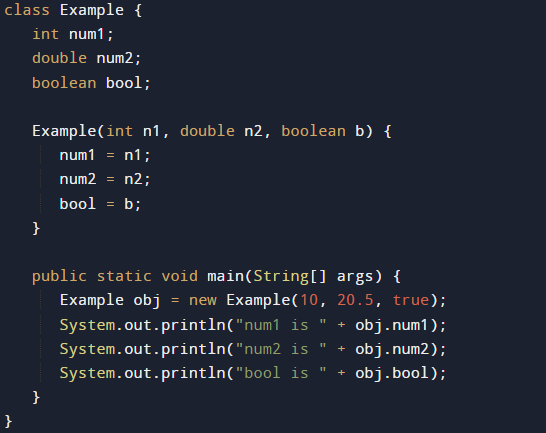
ii) Copy Constructors:
Shallow copy constructor: A shallow copy constructor is a constructor that creates a new object with the same values as the original object. In a shallow copy, only the values of the primitive data types are copied, while the reference variables are copied as a reference to the original object.
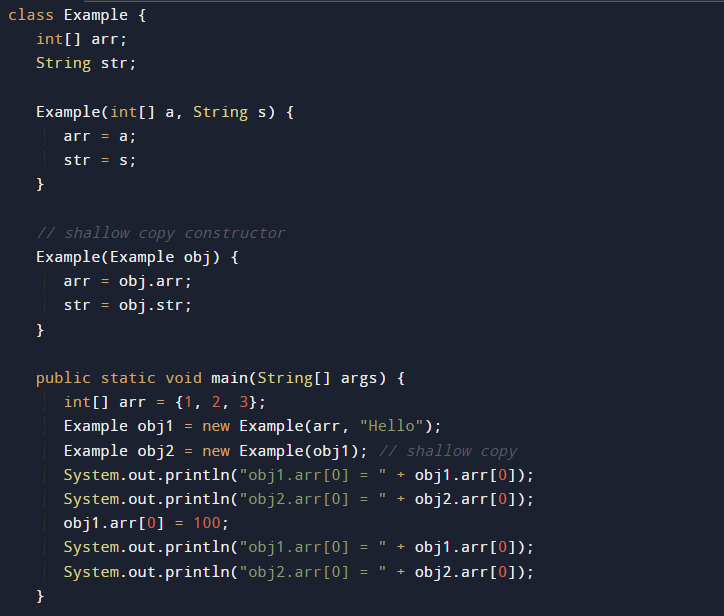
Deep copy constructor: A deep copy constructor is a constructor that creates a new object with the same values as the original object. In a deep copy, both the primitive data types and reference variables are copied as new objects.
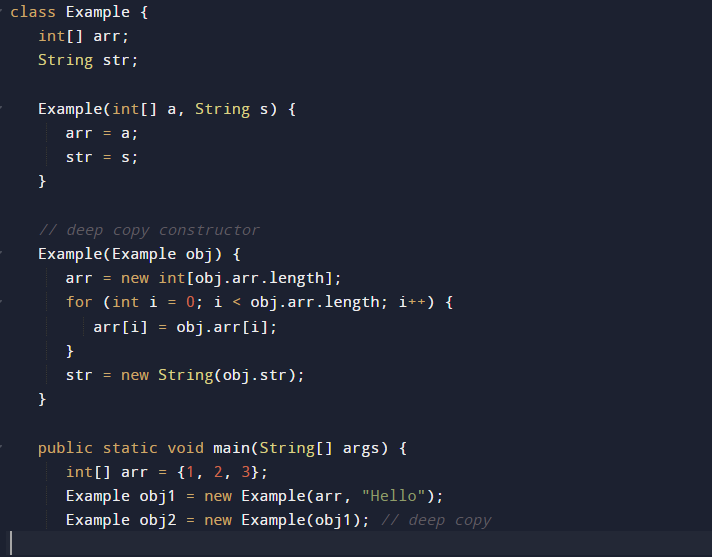
(c) Explain file io using byte stream with appropriate example.
hint: use FileInputStream, FileOutputStream
File input/output (I/O) is a mechanism to read/write data to/from files in a computer’s storage system. Java provides two types of streams for file I/O operations: byte stream and character stream. In this answer, we will explain file I/O using byte stream with an appropriate example.
Byte stream operates with raw bytes and is used for handling binary data like images, audio files, etc. Java provides FileInputStream and FileOutputStream classes to handle file I/O using byte streams. FileInputStream reads data from a file as a stream of bytes, while FileOutputStream writes data to a file as a stream of bytes.
Here is an example program that demonstrates file I/O using byte stream:
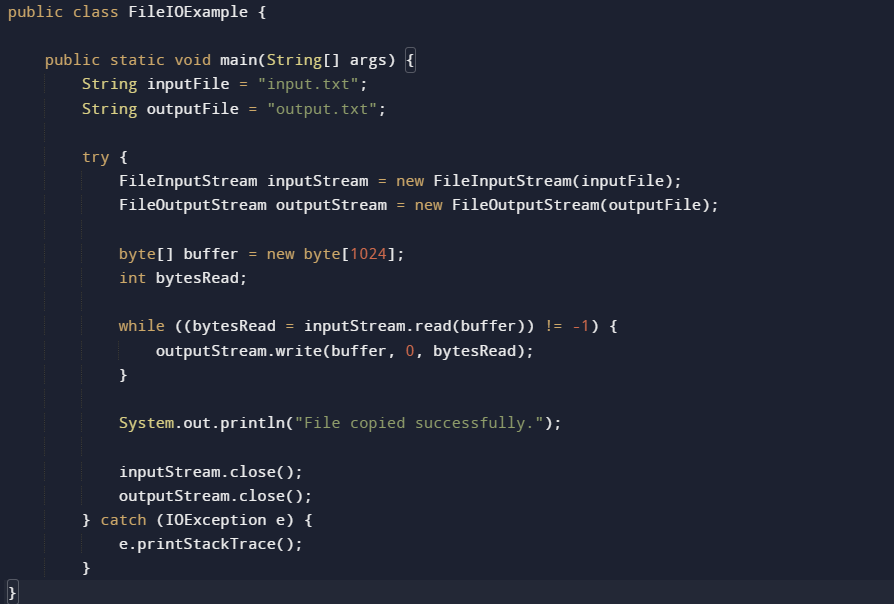
The program first creates FileInputStream and FileOutputStream objects for the input and output files, respectively.
Then, it creates a byte array buffer of size 1024 bytes to read data from the input file in chunks. The read()
method of FileInputStream
is used to read data into the buffer, which returns the number of bytes read. The while loop runs until all the data is read from the input file.
The write()
method of FileOutputStream is used to write data from the buffer to the output file. The first argument of write()
is the buffer, the second argument is the starting index from where to write data, and the third argument is the number of bytes to write. The program closes both the input and output streams after the file copy is completed.
(a) Define types of polymorphism
Polymorphism is the ability of an object to take on many forms. In Java, there are two types of polymorphism:
Compile-time polymorphism (or Static polymorphism): It is achieved through method overloading or operator overloading. In this type, the method to be called is resolved at compile-time based on the number, type, and order of the arguments passed to it. The compiler selects the appropriate overloaded method during compilation.
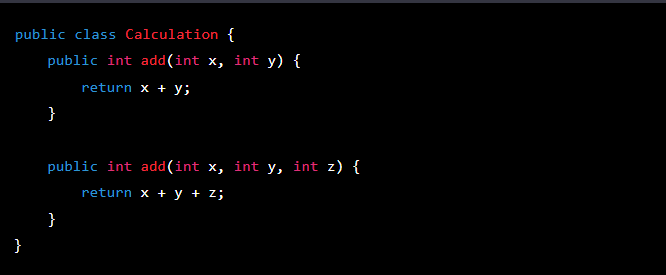
In this example, the Calculation class has two methods with the same name, but different numbers of arguments. The add()
method is overloaded with two and three integer arguments. The method to be called is determined at compile-time based on the number of arguments passed.
Runtime polymorphism (or Dynamic polymorphism): It is achieved through method overriding. In this type, the method to be called is resolved at runtime based on the actual object that is referenced (not the reference type). This means that the method to be executed is decided during runtime by the JVM, depending on the type of the object that is created.
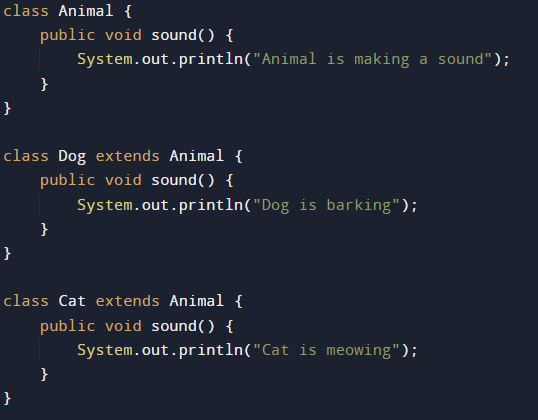
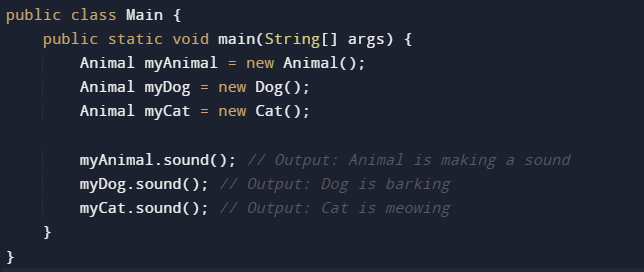
In this example, we have an Animal
class with a sound()
method, which is overridden in the Dog
and Cat
classes. During runtime, the JVM decides which sound()
method to call based on the actual object that is referenced, not the reference type. In the main method, we create three objects: myAnimal
, myDog
, and myCat
. When we call the sound()
method on these objects, the method that is executed depends on the type of object that is created. This is an example of runtime polymorphism.
(b) Explain the following:
i) Arguments and Parameters of a function
ii) Pass by Value and Pass by reference
i) Arguments and Parameters of a function: In Java, a method or function can have parameters and arguments. Parameters are the variables declared in a method’s declaration whereas, arguments are the values that are passed to the parameters during the method call.
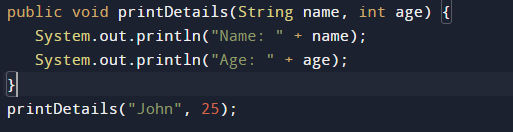
Here, name
and age
are the parameters of the method printDetails()
, and while calling this method, we can pass the values to these parameters as arguments, like:
ii) Pass by Value and Pass by reference: In Java, the arguments passed to a method can be passed either by value or by reference.
Pass by value
means that a copy of the value of the argument is passed to the method, and any changes made to the parameter inside the method does not affect the original value of the argument outside the method.Pass by reference
means that the actual reference to the object is passed to the method, and any changes made to the object inside the method affects the original object outside the method.
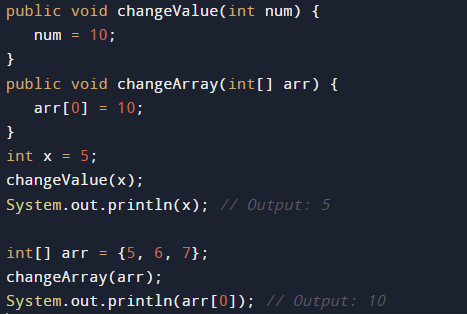
Here, x
is passed as an argument to the method changeValue()
by value, and the parameter num
inside the method is assigned a new value of 10
. But this change in the parameter does not affect the original value of x
outside the method, and hence the output is 5
.
and arr
is passed as an argument to the method changeArray()
by reference, and the parameter arr
inside the method is used to change the first element of the array to 10
. This change in the parameter affects the original array outside the method, and hence the output is 10
.
(c) Explain file io using character stream with appropriate example.
hint: use FileReader, FileWriter
File IO using character streams in Java is a way of reading and writing data to and from a file in a character-wise manner. This is useful when we need to deal with text-based data that contains special characters such as newline, tab, or any non-ASCII characters. Java provides two classes – FileReader and FileWriter – to perform file IO operations using character streams.
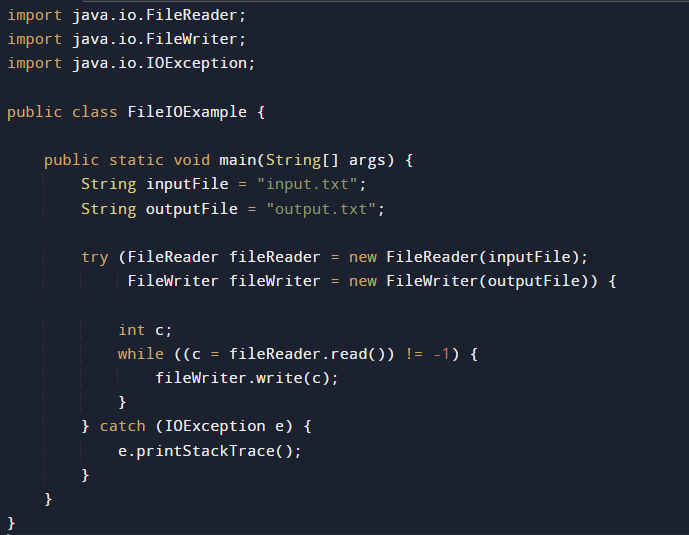
In this example, we’re reading from a file called input.txt
and writing to another file called output.txt
. We use a try-with-resources
block to ensure that the FileReader
and FileWriter
objects are properly closed after we’re done using them.
Inside the try
block, we’re using the read()
method of FileReader
to read the file character by character. This method returns -1
when the end of the file is reached. We’re then using the write()
method of FileWriter
to write the read characters to the output file.
Note that we’re not using any buffer to read or write the data. This is because the FileReader
and FileWriter
classes automatically use a buffer internally for performance optimization.
(a) Define Encapsulation and access specifier
Encapsulation is a mechanism in object-oriented programming that combines data and functions that operate on that data into a single unit called a class. It allows us to hide the implementation details of an object from the outside world and restrict access to its internal state.
Access specifiers, also known as access modifiers, determine the level of access to the members of a class in Java. There are four access specifiers in Java:
- Public: Members declared as public are accessible from anywhere in the program.
- Private: Members declared as private are only accessible within the same class.
- Protected: Members declared as protected are accessible within the same class, within its subclasses, and within other classes in the same package.
- Default: Members declared with no access specifier (i.e., no public, private, or protected keyword) are accessible within the same package.
The use of access specifiers is important for encapsulation, as it allows us to control the visibility of a class’s members and prevent external code from modifying its internal state directly.
(b) Explain multithreading using Thread class
Multithreading is a feature in Java that allows multiple threads to run concurrently within a single program. This means that different parts of the program can execute independently and simultaneously. In Java, multithreading is achieved using the Thread
class.
The Thread
class represents a thread of execution. To use threads, you can either extend the Thread
class or implement the Runnable
interface. Here’s an example of using the Thread
class:
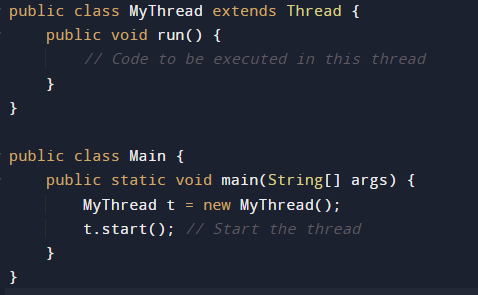
In this example, we create a new class MyThread
that extends the Thread
class. We override the run()
method of the Thread
class with the code that we want to execute in this thread. Then in the main()
method, we create an instance of MyThread
and start it using the start()
method. This starts the execution of the run()
method in a new thread.
(c) Write a short note on Java Collections.
In Java, a collection is a group of objects that can be treated as a single entity. Java provides a framework of classes and interfaces called the Java Collections Framework that makes it easy to work with collections of objects.
The Java Collections Framework provides several interfaces and classes that represent different types of collections, such as lists, sets, maps, and queues. Each collection type has its own set of characteristics and can be used for different purposes.
For example, a List
is an ordered collection that allows duplicates, while a Set
is an unordered collection that does not allow duplicates. A Map
is a collection that maps keys to values, and a Queue
is a collection that represents a waiting line or a sequence of elements to be processed in a particular order.
(a) Differentiate between Abstract class and Interfaces
Abstract Class | Interface |
---|---|
An abstract class can have both abstract and non-abstract methods. | An interface can only have abstract methods. |
An abstract class can have instance variables. | An interface cannot have instance variables. |
An abstract class can have constructors. | An interface cannot have constructors. |
An abstract class can be extended by only one class. | An interface can be implemented by multiple classes. |
An abstract class can have access modifiers for its methods and variables. | All methods and variables in an interface are public by default. |
An abstract class can be used to define default behavior for subclasses. | An interface is used to define a contract for implementing classes to follow. |
An abstract class can have static and non-static methods. | All methods in an interface are implicitly abstract and cannot be static. |
(b) Explain multithreading using Runnable interface
In Java, multithreading can be implemented using the Runnable interface. The Runnable interface
provides a way to define a thread’s run()
method, which contains the code to be executed by the thread.
To create a new thread using the Runnable interface, we need to follow these steps:
- Create a class that implements the
Runnable interface
. - Override the
run()
method in the class with the code to be executed by the thread. - Create an instance of the class.
- Create a new Thread object and pass the instance of the class as an argument to the constructor.
- Call the
start()
method on the Thread object to start the thread.
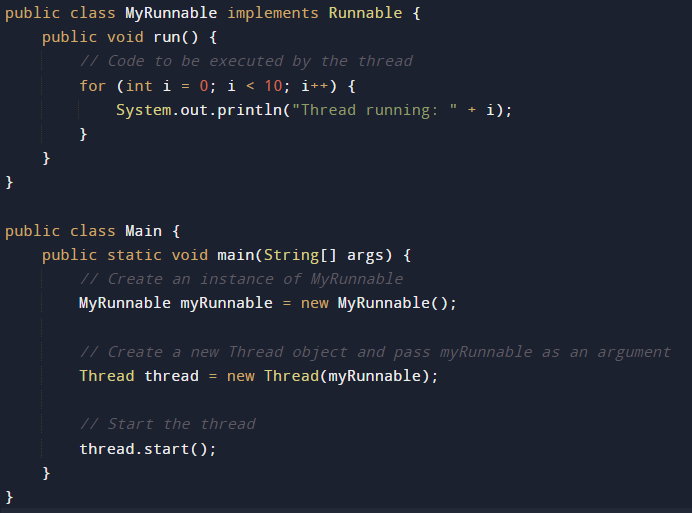
(c) Write a program to add input elements in ArrayList collection class,
then sort the inserted elements in descending order and display the
sorted output.
hint: use Collections.reverseOrder()
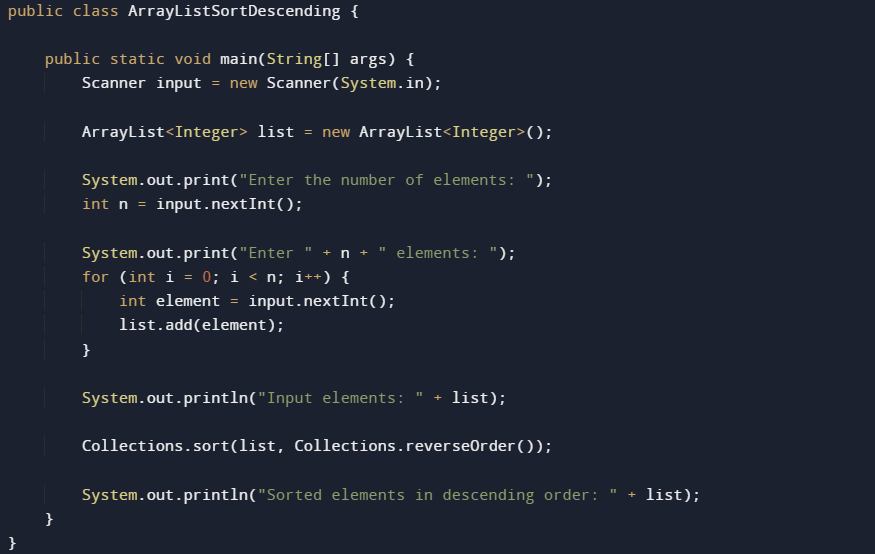
Explanation:
- Create a
Scanner
object to read input from the user. - Create an
ArrayList
object to store the input elements. - Prompt the user to enter the number of elements to be added to the list.
- Prompt the user to enter the elements one by one and add them to the list.
- Display the input elements using
System.out.println()
method. - Sort the elements in descending order using the
Collections.sort()
method andCollections.reverseOrder()
comparator. - Display the sorted elements using
System.out.println()
method.
(a) Explain following Java keywords using appropriate examples:
i) throw, ii) throws, iii) finally
i) throw
is a Java keyword used to explicitly throw an exception. It is used to throw a new exception or re-throw an existing exception to the calling method or higher up in the call stack. Here is an example:
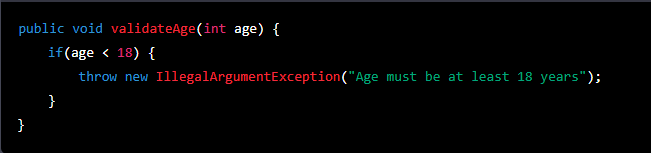
ii) throws
is a Java keyword used to declare that a method may throw one or more checked exceptions. It is used in the method signature to specify the exceptions that a method can throw but does not handle. Here is an example:
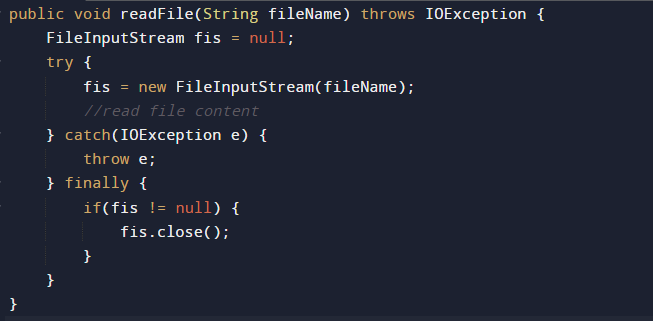
iii) finally
is a Java keyword used to create a block of code that will be executed after a try/catch block, regardless of whether an exception is thrown or not. It is used to release resources or perform clean-up operations. Here is an example:
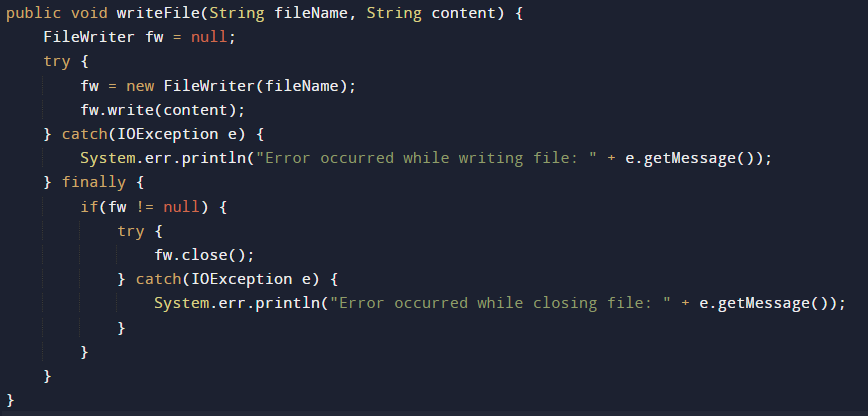
(b) In multi-threading using Thread class, explain with an example how
a start() method call invokes the run method of the class extending
Thread class.
In Java, the Thread
class is used to create and control threads of execution. Each thread in Java is associated with an instance of the Thread
class. To create a new thread, we can either create a class that extends the Thread
class or implement the Runnable
interface.
When we create a class that extends the Thread
class, we must override the run()
method. The run()
method contains the code that the thread will execute when it starts. To start the thread, we call the start()
method on the Thread
instance. This method will create a new thread of execution and then call the run()
method on the new thread.
Here is an example of creating a class that extends the Thread
class and overriding the run()
method:
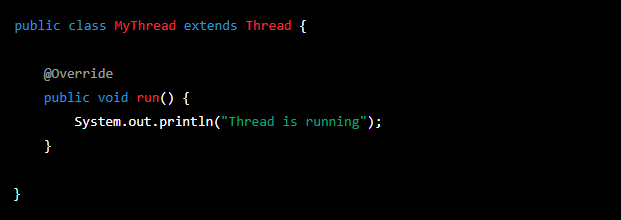
To start the thread, we can create an instance of the MyThread
class and call the start()
method:
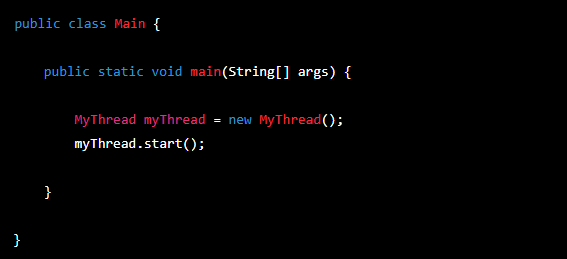
When we call myThread.start()
, a new thread of execution is created and the run()
method is called on the new thread. The output of the above code will be:
Output:
Thread is running
(c) Write a short note on JAVAFX controls.
JavaFX is a framework for creating rich client applications using Java. It provides a set of controls that can be used to create user interfaces for desktop and mobile applications. These controls are built on top of the JavaFX scene graph and can be customized using CSS.
JavaFX controls are designed to be easy to use and provide a rich set of features. Some of the commonly used JavaFX controls include:
Button
: A button is a control that can be clicked to perform an action.Label
: A label is a control that displays text.TextField
: A text field is a control that allows the user to enter text.TextArea
: A text area is a control that allows the user to enter multiple lines of text.CheckBox
: A check box is a control that allows the user to select or deselect a value.RadioButton
: A radio button is a control that allows the user to select one option from a set of options.ComboBox
: A combo box is a control that allows the user to select one value from a drop-down list.ListView
: A list view is a control that displays a list of items.TableView
: A table view is a control that displays data in a tabular format.DatePicker
: A date picker is a control that allows the user to select a date from a calendar.
JavaFX controls can be added to a scene graph and can be customized using CSS. They provide a rich set of features, including event handling, validation, and data binding. JavaFX controls are also highly customizable and can be styled to match the look and feel of your application. Overall, JavaFX controls provide a powerful and flexible way to create rich client applications using Java.
(a) Explain thread life cycle
The life cycle of a thread in Java can be described using the following diagram:
- New: The thread is in the new state when it is created, but it has not yet started to execute.
- Runnable: When the start() method is called on a thread object, it enters the runnable state. In this state, the thread is ready to run, but the operating system has not yet assigned a processor to it.
- Running: When the operating system assigns a processor to the thread, it enters the running state. The thread executes the code in its run() method.
- Blocked: A thread enters the blocked state when it is waiting for a monitor lock to be released, such as when it is waiting to enter a synchronized block.
- Waiting: A thread enters the waiting state when it is waiting for another thread to perform a particular action, such as when it calls the wait() method.
- Timed Waiting: A thread enters the timed waiting state when it is waiting for a specified period of time, such as when it calls the sleep() method.
- Terminated: When a thread completes its run() method or throws an unhandled exception, it enters the terminated state and cannot be restarted.
This life cycle diagram of a thread in Java illustrates how a thread can transition from one state to another based on various conditions and events.
(b) In multi-threads using the Runnable interface, explain with an
example how a start() method calls the run() method of a class
implementing a runnable interface.
In Java, the Thread
class is used to create and control threads of execution. Each thread in Java is associated with an instance of the Thread
class. To create a new thread, we can either create a class that extends the Thread
class or implement the Runnable
interface.
When we create a class that extends the Thread
class, we must override the run()
method. The run()
method contains the code that the thread will execute when it starts. To start the thread, we call the start()
method on the Thread
instance. This method will create a new thread of execution and then call the run()
method on the new thread.
Here is an example of creating a class that extends the Thread
class and overriding the run()
method:
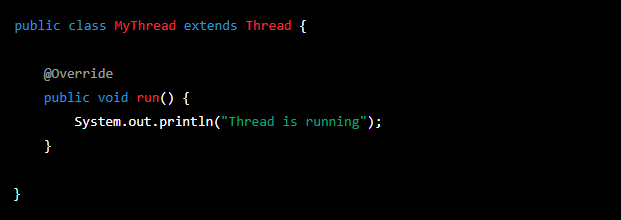
To start the thread, we can create an instance of the MyThread
class and call the start()
method:
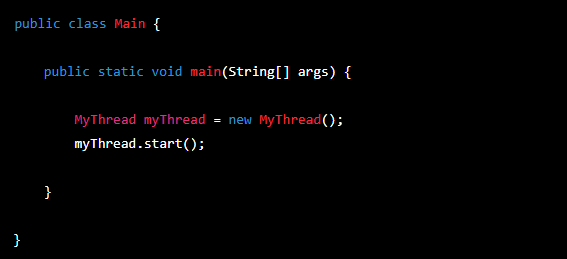
When we call myThread.start()
, a new thread of execution is created and the run()
method is called on the new thread. The output of the above code will be:
Output:
Thread is running
(c) Develop a GUI based application using JAVAFX controls.
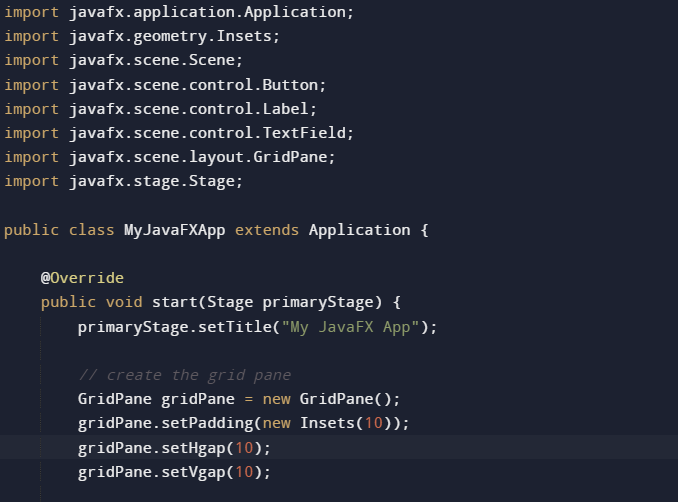
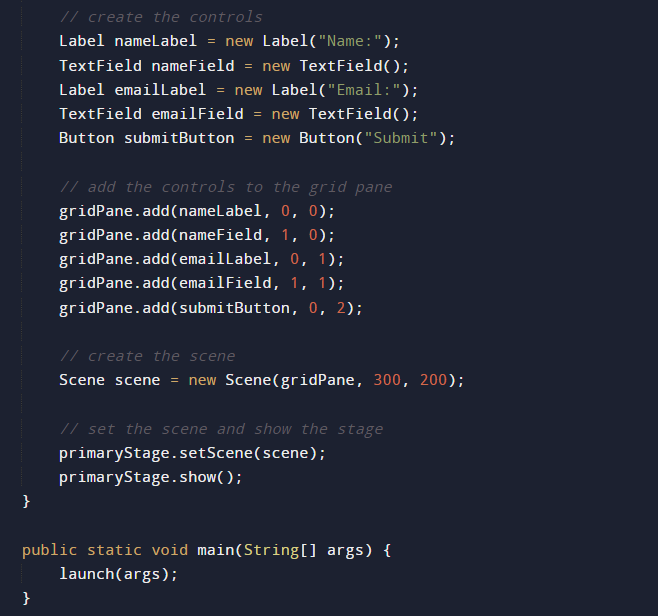
Read More : OOP GTU Paper Solution Winter 2021
Read More : DS GTU Paper Solution Winter 2021
“Do you have the answer to any of the questions provided on our website? If so, please let us know by providing the question number and your answer in the space provided below. We appreciate your contributions to helping other students succeed.